top of page
All of us do not have equal talent. But, all of us have an equal opportunity to develop our talents.
-A.P.J Abdul Kalam
Search
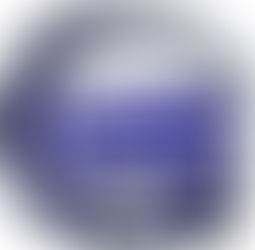
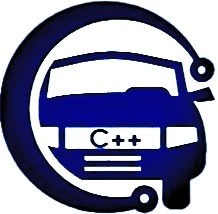
Reverse an array in same array.
#include <iostream> using namespace std; int main() { int arr[] = {1,2,3,4,5,6,7}; int len = sizeof(arr)/sizeof(arr[0]); for(int i =...
Apr 21, 20221 min read
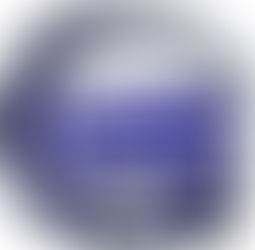
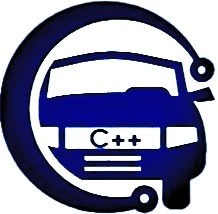
write a program to reverse word in a string.
#include <iostream> //#include<string.h> #include<string> using namespace std; int main() { //char str[100] = {"Hello World"}; string str...
Mar 31, 20221 min read
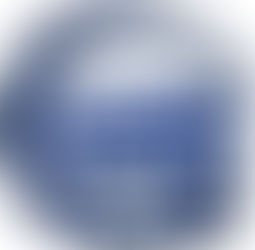
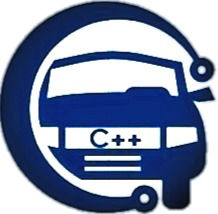
Producer Consumer Program in Multithreading.
#include <iostream> #include<deque> #include<thread> #include<mutex> #include<condition_variable> std::deque<int> buffer; std::mutex mu;...
Mar 27, 20221 min read
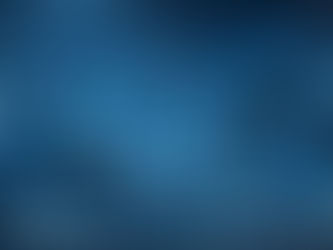
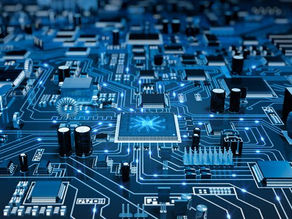
Count number of bits to be flipped to convert a to b.
#include <iostream> using namespace std; int main() { int a = 3 , b = 4; int count = 0; int x = a ^ b; cout<<x<<endl; while(x) { if(x...
Mar 18, 20221 min read
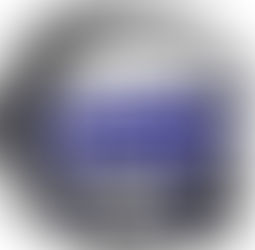
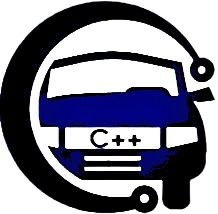
Print the odd/even number through multiple thread.
// Example program #include <iostream> #include<thread> #include<mutex> #include<condition_variable> #include<chrono> using namespace...
Jan 29, 20221 min read
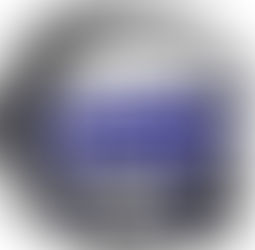
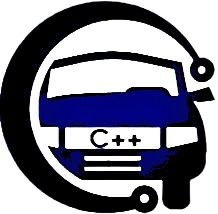
sort array
// Example program #include<iostream> using namespace std; void sort(int *arr,int length) { for(int i =0; i<length;i++) { for(int j =...
Jan 28, 20221 min read
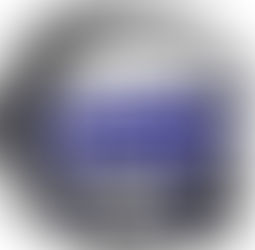
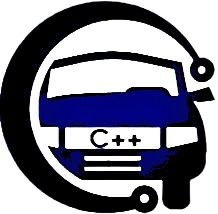
How to Find the 2nd Highest number in array.
// Example program #include<iostream> using namespace std; int getMaxValue(int *arr,int length) { int max = arr[0]; int max1 = arr[0];...
Jan 28, 20221 min read
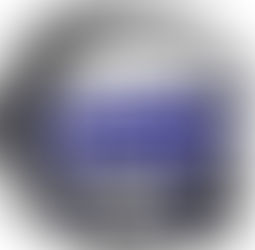
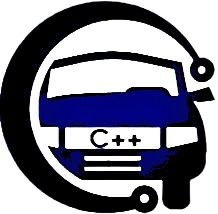
Reverse Single LinkedList using class
#include<iostream> using namespace std; class node { public: int info; node *next; }; class list { private: node *head; public: list()...
Dec 12, 20211 min read
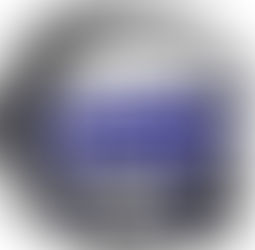
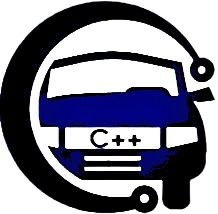
Create Single LinkedList using class.
#include<iostream> using namespace std; class node { public: int info; node *next; }; class list { private: node *head; public: list()...
Dec 12, 20211 min read
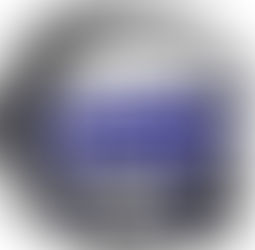
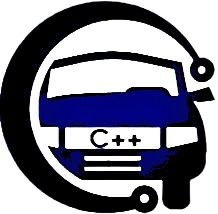
Move constructor in c++.
rvalue Reference: rvalue references can do what lvalue references fails to do i.e. a rvalue reference can refer to rvalues. Declaring...
Jul 4, 20213 min read
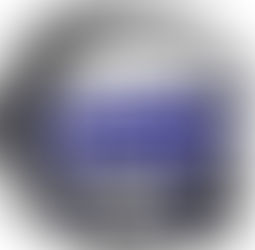
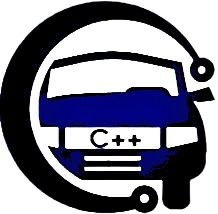
Virtual function and Internal Implementation.
A virtual function is a member function which is declared within a base class and is re-defined(Overriden) by a derived class. Virtual...
May 30, 20213 min read
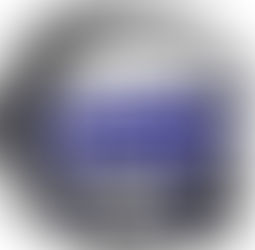
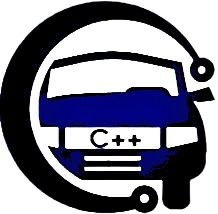
Stack Implementation using class/LinkedList.
Stack: A stack is an abstract data structure that contains a collection of elements. He order may be LIFO(Last In First Out) or...
May 27, 20212 min read
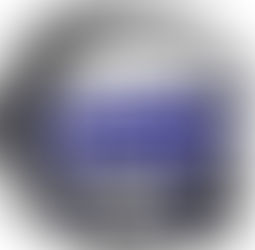
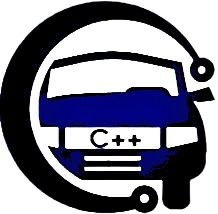
C++ 14 Features.
C++14 is a version for the programming language C++. New language features Function return type deduction Alternate type deduction on...
May 23, 20211 min read
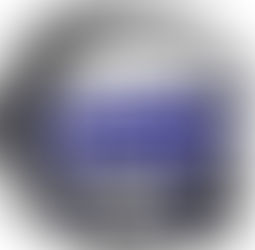
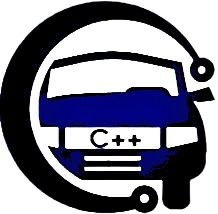
Singly Linked List.
Singly Linked List operation through structure: #include<iostream> using namespace std; typedef struct nodetype { int info; struct...
May 15, 20212 min read
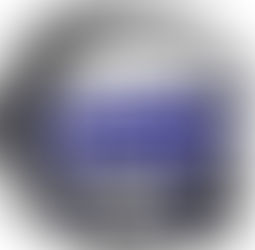
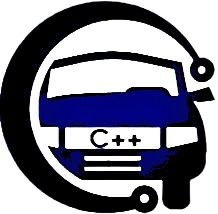
Search Operation
Searching is the process of finding the location of given element in the array. The search is said to be successful if the given element...
May 14, 20211 min read
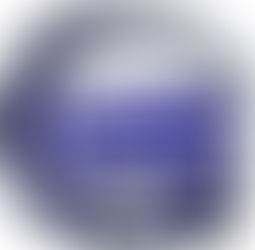
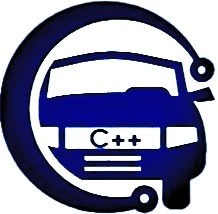
Design Pattern
what is design pattern? Design patterns are typical solutions to commonly occurring problems in software design. They are like pre-made...
May 9, 20211 min read
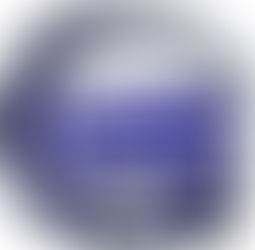
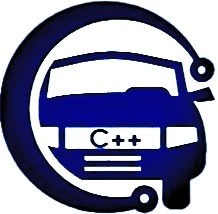
constant pointer and pointer to constant in C++
Constant Pointer: Constant pointer is a pointer that can not change the address it holding. Hence once a constant pointer points to a...
May 2, 20211 min read
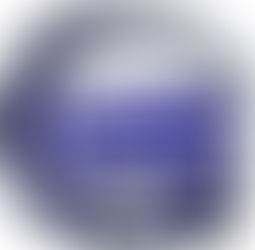
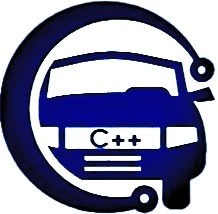
Pointer and Reference in C++
1> what is deference between pointer and reference? Pointer: Pointer is variable which store the address of the another variable. syntax:...
May 1, 20212 min read
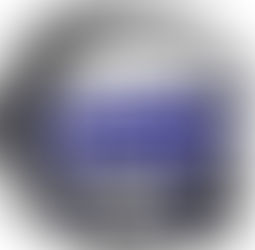
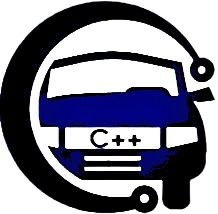
Lambda Function in C++
Lambda functions are a kind of anonymous functions in C++. These are mainly used as callbacks in C++. Lambda function is like a normal...
Apr 29, 20211 min read
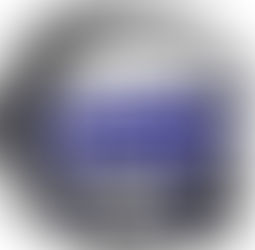
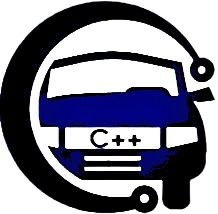
Sequence containers in C++
Sequence containers implement data structures which can be accessed sequentially. array: Static contiguous array (class template)...
Apr 13, 20212 min read
bottom of page