Print the odd/even number through multiple thread.
- prashant raj
- Jan 29, 2022
- 1 min read
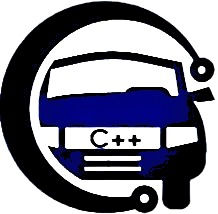
// Example program
#include <iostream>
#include<thread>
#include<mutex>
#include<condition_variable>
#include<chrono>
using namespace std;
std::mutex m;
std::condition_variable cv1,cv2;
void evenDisplay()
{
std::unique_lock<mutex> lck(m);
for(int i=0;i<50;i++)
{
if((i & 1)==0)
{
cout<<"even Thread:"<<i<<endl;
cv1.wait(lck);
}
cv2.notify_one();
}
}
void oddDisplay()
{
std::unique_lock<mutex> lck(m);
for(int i=0;i<50;i++)
{
if((i & 1)==1)
{
cout<<"odd Thread:"<<i<<endl;
cv2.wait(lck);
}
cv1.notify_one();
}
}
int main()
{
thread t1(evenDisplay);
std::this_thread::sleep_for(std::chrono::milliseconds(1));
thread t2(oddDisplay);
t1.join();
t2.join();
}
Comentarios