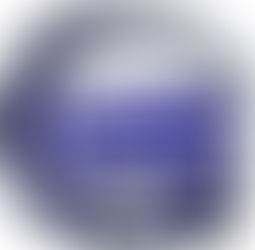
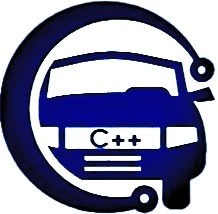
Reverse an array in same array.
#include <iostream> using namespace std; int main() { int arr[] = {1,2,3,4,5,6,7}; int len = sizeof(arr)/sizeof(arr[0]); for(int i =...
prashant raj
Apr 21, 20221 min read
6
0
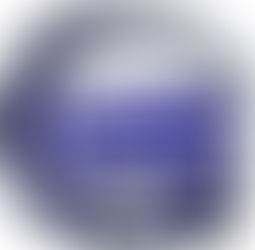
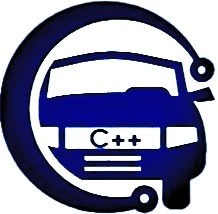
write a program to reverse word in a string.
#include <iostream> //#include<string.h> #include<string> using namespace std; int main() { //char str[100] = {"Hello World"}; string str...
prashant raj
Mar 31, 20221 min read
9
0
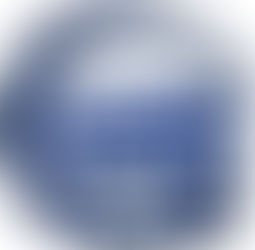
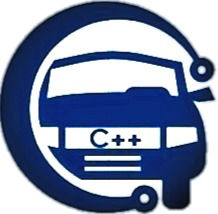
Producer Consumer Program in Multithreading.
#include <iostream> #include<deque> #include<thread> #include<mutex> #include<condition_variable> std::deque<int> buffer; std::mutex mu;...
prashant raj
Mar 27, 20221 min read
9
0
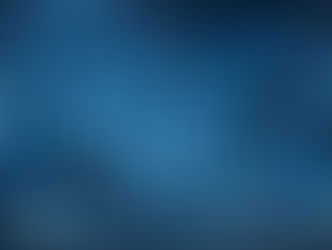
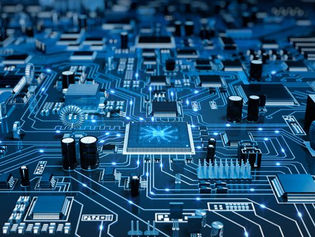
Write a program to find whether a number is power of 2?
#include <iostream> using namespace std; int main() { int a = 128; int b = a-1; if(a && !(a & b)) { cout<<"Number is power of 2"<<endl;...
prashant raj
Mar 18, 20221 min read
7
0
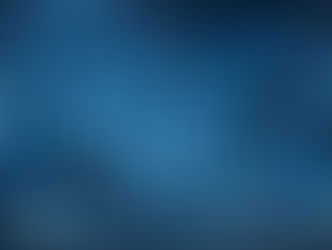
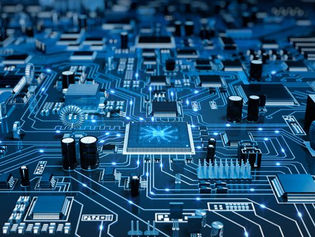
Count number of bits to be flipped to convert a to b.
#include <iostream> using namespace std; int main() { int a = 3 , b = 4; int count = 0; int x = a ^ b; cout<<x<<endl; while(x) { if(x...
prashant raj
Mar 18, 20221 min read
10
0
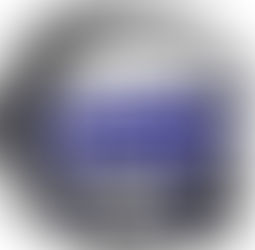
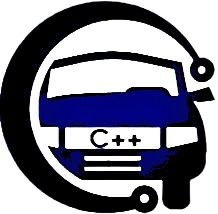
String
Reverse the String through character pointer. #include<iostream> #include<string.h> using namespace std; void reverse(char *ptr1) { int...
prashant raj
Feb 3, 20221 min read
10
0
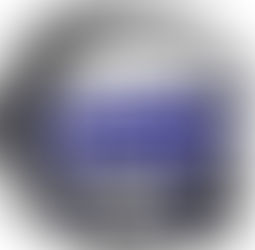
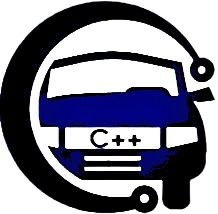
Print the odd/even number through multiple thread.
// Example program #include <iostream> #include<thread> #include<mutex> #include<condition_variable> #include<chrono> using namespace...
prashant raj
Jan 29, 20221 min read
22
0
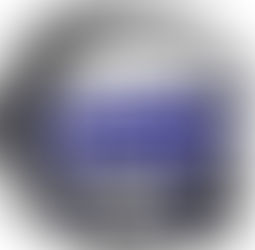
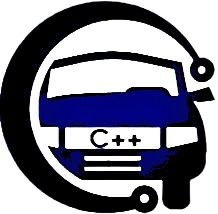
sort array
// Example program #include<iostream> using namespace std; void sort(int *arr,int length) { for(int i =0; i<length;i++) { for(int j =...
prashant raj
Jan 28, 20221 min read
7
0
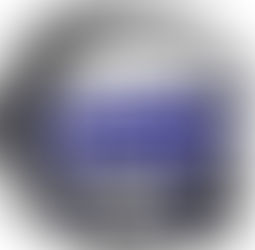
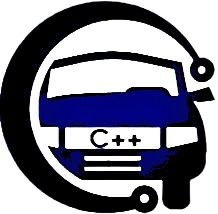
How to Find the 2nd Highest number in array.
// Example program #include<iostream> using namespace std; int getMaxValue(int *arr,int length) { int max = arr[0]; int max1 = arr[0];...
prashant raj
Jan 28, 20221 min read
9
0
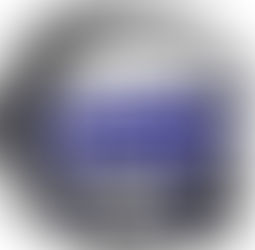
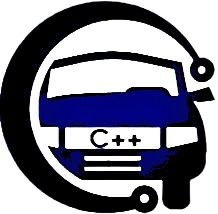
Reverse Single LinkedList using class
#include<iostream> using namespace std; class node { public: int info; node *next; }; class list { private: node *head; public: list()...
prashant raj
Dec 12, 20211 min read
14
0