Producer Consumer Program in Multithreading.
- prashant raj
- Mar 27, 2022
- 1 min read
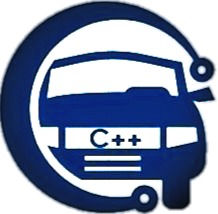
#include <iostream>
#include<deque>
#include<thread>
#include<mutex>
#include<condition_variable>
std::deque<int> buffer;
std::mutex mu;
std::condition_variable cv;
using namespace std;
int bufferSize = 50;
void producer(int val)
{
while(val)
{
std::unique_lock<std::mutex> locker(mu);
cv.wait(locker,[](){ return buffer.size() < bufferSize;});
buffer.push_back(val);
cout<<"producer produced"<<val<<endl;
val--;
locker.unlock();
cv.notify_one();
}
}
void consumer()
{
while(true)
{
std::unique_lock<std::mutex> locker(mu);
cv.wait(locker,[](){ return buffer.size() > 0;});
int value = buffer.back();
buffer.pop_back();
cout<<"consumer consumed"<<value<<endl;
locker.unlock();
cv.notify_one();
}
}
int main()
{
thread t1(producer,50);
thread t2(consumer);
t1.join();
t2.join();
return 0;
}
Comments