Sequence containers in C++
- prashant raj
- Apr 13, 2021
- 2 min read
Updated: May 2, 2021
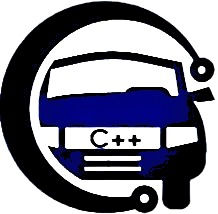
Sequence containers implement data structures which can be accessed sequentially.
array: Static contiguous array (class template)
#include<iostream>
#include<array>
using namespace std;
int main()
{
array<int,6> arr= {3,6,8,9,3,2};
array<int,6>::iterator itr;
//general method
/*for(int i=0 ; i<arr.size();i++ )
{
cout<<arr.at(i)<<endl;
}*/
//iterator method
for(itr=arr.begin();itr!=arr.end();itr++)
{
cout<<*itr<<endl;
}
return 0;
}
Output:- 3
6
8
9
3
2
vector: Dynamic contiguous array (class template)
Dynamic contiguous array (class template)
Vectors are sequence containers representing arrays that can change in size.
Just like arrays, vectors use contiguous storage locations for their elements, which means that their elements can also be accessed using offsets on regular pointers to its elements, and just as efficiently as in arrays. But unlike arrays, their size can change dynamically, with their storage being handled automatically by the container.
#include<iostream>
#include<vector>
using namespace std;
int main()
{
vector<int> v1;
vector<int> v2;
vector <int> v3;
int myints[] = {1776,7,4};
v3.assign (myints,myints+3);
v2.assign(7,100);
vector<int>::iterator itr;
for(int i=1;i<=10;i++)
{
v1.push_back(i*10);
}
v1.insert(v1.begin()+3,15);
v1.emplace(v1.begin(),25);
for(itr = v1.begin();itr!=v1.end();itr++)
{
cout<<*itr<<endl;
}
for(int i=0 ;i<v2.size();i++)
{
cout<<v2.at(i)<<endl;
}
for(int i=0 ;i<v3.size();i++)
{
cout<<v3.at(i)<<endl;
}
}
deque: Double-ended queue (class template)
Deque is a double ended queue container that provides insertion and deletion at both ends i.e. front and back with.
A deque is generally implemented as a collection of memory blocks. These memory blocks contains the elements at contiguous locations.
#include<iostream>
#include<deque>
using namespace std;
int main()
{
deque<int> mydeq;
mydeq.push_back(10);
mydeq.push_back(20);
mydeq.push_front(15);
deque<int>::iterator itr;
for(itr=mydeq.begin();itr!=mydeq.end();itr++)
{
cout<<*itr<<endl;
}
mydeq.push_back(0);
mydeq.pop_front();
if(mydeq.back()!=0)
{
cout<<"Zero is not present at end"<<endl;
}
else
cout<<"Zero is present at end"<<endl;
for(itr=mydeq.begin();itr!=mydeq.end();itr++)
{
cout<<*itr<<endl;
}
mydeq.clear();
cout<<"Size of deque is "<<mydeq.size()<<endl;
}
forward_list: Singly-linked list (class template)
list : Doubly-linked list (class template)
Lists are sequence containers that allow constant time insert and erase operations anywhere within the sequence, and iteration in both directions as we as also that allow non-contiguous memory allocation. As compared to vector, list has slow traversal, but once a position has been found, insertion and deletion are quick. Normally, when we say a List, we talk about doubly linked list.
Modifiers: assign Assign new content to container (public member function ) emplace_front Construct and insert element at beginning (public member function ) push_front Insert element at beginning (public member function ) pop_front Delete first element (public member function ) emplace_back Construct and insert element at the end (public member function ) push_back Add element at the end (public member function ) pop_back Delete last element (public member function ) emplace Construct and insert element (public member function ) insert Insert elements (public member function ) erase Erase elements (public member function ) swap Swap content (public member function ) resize Change size (public member function ) clear Clear content (public member function )
#include<iostream>
#include<list>
using namespace std;
int main()
{
list<int> mylist;
mylist.push_back(10);
mylist.assign(3,20);
list<int>::iterator itr;
itr = mylist.begin();
itr++;
mylist.insert(itr,15);
mylist.insert(itr,3,18);
for(itr = mylist.begin();itr!=mylist.end();itr++)
{
cout<<*itr<<endl;
}
return 0;
}
Output:
20
15
18
18
18
20
20
Comentários