String
- prashant raj
- Feb 3, 2022
- 1 min read
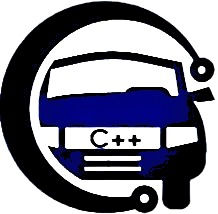
Reverse the String through character pointer.
#include<iostream>
#include<string.h>
using namespace std;
void reverse(char *ptr1)
{
int length = strlen(ptr1);
char *temp = new char[length];
for(int i=length-1;i>=0;i--)
{
static int j =0;
temp[j] = ptr1[i];
j++;
}
cout<<temp<<endl;
}
int main()
{
char *ptr = "Hello";
reverse(ptr);
return 0;
}
Reverse the string through character array.
#include<iostream>
#include<string.h>
using namespace std;
void reverse(char ptr1[])
{
int length = strlen(ptr1);
char *temp = new char[length];
for(int i=length-1;i>=0;i--)
{
static int j =0;
temp[j] = ptr1[i];
j++;
}
cout<<temp<<endl;
}
int main()
{
char ptr[] = "Hello";
reverse(ptr);
return 0;
}
Reverse the string through string class.
Reverse the string through the string class.
// Example program
#include<iostream>
#include<string>
using namespace std;
void reverseString(string &str)
{
string temp = str;
int length = str.length();
for(int i = length-1;i>=0;i--)
{
static int j =0;
str[j] = temp[i];
j++;
}
}
int main()
{
string str = "Prashant";
reverseString(str);
cout<<str<<endl;
}
swap string through character array.
#include<iostream>
#include<string.h>
using namespace std;
void swap(char *temp,char *temp1)
{
int length = strlen(temp);
char *temp2 = new char[length];
strcpy(temp2,temp);
strcpy(temp,temp1);
strcpy(temp1,temp2);
}
int main()
{
char str[] = "Hello";
char str1[] = "Bolo";
cout<<str<<endl;
cout<<str1<<endl;
swap(str,str1);
cout<<str<<endl;
cout<<str1<<endl;
}
Comments