STL in C++
- prashant raj
- Apr 2, 2021
- 2 min read
Updated: May 2, 2021
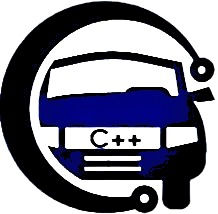
The Standard Template Library (STL) is a set of C++ template classes to provide common programming data structures and functions such as lists, stacks, arrays, etc. It is a library of container classes, algorithms, and iterators.
STL has four Components:
· Algorithms
· Containers
· Functions
· Iterators
Algorithms: Algorithm defines a collection of functions especially designed to be used on ranges of elements.They act on containers and provide means for various operations for the contents of the containers.
Containers: Containers or container classes store objects and data.
Types of Containers
Sequence Containers: implement data structures which can be accessed in a sequential manner. Like as:vector, list, deque, array etc
Container Adaptors: provide a different interface for sequential containers.
Like as:queue,priority_queue,stack etc.
container adapters—such as stack, queue, and priority_queue—apply some sort of constraints in the storage and retrieval process of elements in the underlying data structure.
Container adapter classes are typically convenient classes provided by the library for everyday programming
Associative Containers: implement sorted data structures that can be quickly searched (O(log n) complexity).
Like as: set, multiset, map, multimap
Unordered Associative Containers: implement unordered data structures that can be quickly searched.
Like as: unordered_set, unordered_multiset, unordered_map, unordered_multimap.
Functions:
The STL includes classes that overload the function call operator. Instances of such classes are called function objects or functors. Functors allow the working of the associated function to be customized with the help of parameters to be passed.
Iterators:
An iterator is an object (like a pointer) that points to an element inside the container. We can use iterators to move through the contents of the container.
Utility Library
The pair container is a simple container defined in <utility> header consisting of two data elements or objects.
Member Function:
make_pair() : This template function allows to create a value pair without writing the types explicitly.
Syntax:
Pair_name = make_pair (value1,value2);
Comments