Smart pointer in C++
- prashant raj
- Mar 25, 2021
- 2 min read
Updated: May 2, 2021
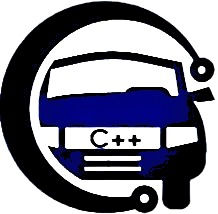
what is smart pointer?
Smart pointer is wrapper class over a pointer with operator like * and -> overloaded.
The object of smart pointer look pointer but can do many things that a normal pointer can’t like automatic destruction ,reference counting and more.
why we use smart pointer?
Using smart pointer we can make pointer to work in a way that we don’t need to explicitly call delete.
// Example program
#include <iostream>
using namespace std;
class smtptr{
int *ptr;
public:
smtptr(int *p = NULL)
{
cout<<"In constructor"<<endl;
ptr = p;
}
int &operator *()
{
return *ptr;
}
int *operator->()
{
return ptr;
}
~smtptr()
{
cout<<"In distructor"<<endl;
delete ptr;
}
};
int main()
{
smtptr smrtptr(new int());
*smrtptr = 20;
cout<<*smrtptr<<endl;
return 0;
}
Generic Smart pointer:
To Create a generic smart pointer we have to use the template functionality.
// Example program
#include <iostream>
using namespace std;
template <class T>
class smtptr{
T *ptr;
public:
smtptr(T *p = NULL)
{
cout<<"In constructor"<<endl;
ptr = p;
}
T &operator *()
{
return *ptr;
}
T *operator->()
{
return ptr;
}
~smtptr()
{
cout<<"In distructor"<<endl;
delete ptr;
}
};
int main()
{
smtptr<int> smrtintptr(new int());
*smrtintptr = 20;
cout<<*smrtintptr<<endl;
smtptr<string> smrtptr(new string());
*smrtptr = "prashant";
cout<<*smrtptr<<endl;
return 0;
}
what is template?
Templates are a feature of the C++ programming language that allows functions and classes to operate with generic types. This allows a function or class to work on many different data types without being rewritten for each one.
C++ adds two new keywords to support templates: ‘template’ and ‘typename’. The second keyword can always be replaced by keyword ‘class’.
why we use template in c++?
if we want to make program generic means that program will work for all the data types.
For example:
a software company may need sort() for different data types. Rather than writing and maintaining the multiple codes, we can write one sort() and pass data type as a parameter.
How templates work?
Templates are expanded at compile time. This is like macros. The difference is, compiler does type checking before template expansion. The idea is simple, source code contains only function/class, but compiled code may contain multiple copies of same function/class.
template <class T>
#include<iostream>
using namespace std;
T maxValue(T x, T y)
{
return (x>y) ? x : y;
}
int main()
{
cout<<maxValue(5,7) <<endl;;
cout<<maxValue(10.5,21.3) <<endl;;
return 0;
}
But at the compilation time compiler will write own code for all the data type like int and float.
So compiler internally generate the below code for data types.
int maxValue (int x,int y)
{
return (x>y) ? x : y;
}
float maxValue (float x, float y)
{
return (x>y) ? x : y;
}
What is the difference between function overloading and templates?
Both function overloading and templates are examples of polymorphism feature of OOP. Function overloading is used when multiple functions do similar operations, templates are used when multiple functions do identical operations.
Types of smart pointer:
Coming Soon.......
Comments