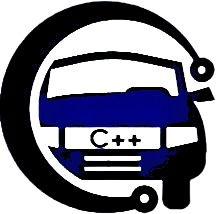
Promise:
promise is a class template and its object promises to set the value in future. Each std::promise object has an associated std::future object that will give the value once set by the std::promise object.
A std::promise object shares data with its associated std::future object
Future:
future is a class template and its object stores the future value.
Actually future object internally store a value that will be assigned in future and it also provide a mechanism to access that value i.e. using get() member function. But if somebody tries to access this associated value of future through get() function before it is available, then get() function will block till value is not available.
#include<iostream>
#include<thread>
#include<future>
using namespace std;
void display(promise <int> *promobj)
{
cout<< "Inside the 2nd thread"<<endl;
promobj->set_value(50);
}
int main()
{
promise <int> promiseobj;
future <int> futureobj = promiseobj.get_future();
thread th1(display,&promiseobj);
cout<<futureobj.get()<<endl;
th1.join();
return 0;
}
Lets understand above example step by step:
Create a std::promise object in Main thread.
std::promise<int> promiseObj;
As of now this promise object doesn’t have any associated value. But it gives a promise that somebody will surely set the value in it and once its set then you can get that value through associated std::future object.
But now suppose Main Thread created this promise object and passed it to Thread 2 object. Now how Main Thread can know that when Thread 2 is going to set the value in this promise object?
The answer is using std::future object.
Every std::promise object has an associated std::future object, through which others can fetch the value set by promise.
So, Main thread will create the std::promise object and then fetch the std::future object from it before passing the std””promise object to thread 2 i.e.
std::future<int> futureObj = promiseObj.get_future();
Now Main Thread will pass the promiseObj to Thread 2.
thread th1(display,&promiseobj);
Then Main thread will fetch the value set by Thread 2 in std::promise through std::future’s get function,
int val = futureObj.get();
But if value is not yet set by thread 2 then this call will get blocked until thread 2 sets the value in promise object i.e.
promiseObj.set_value(45);
If std::promise object is destroyed before setting the value the calling get() function on associated std::future object will throw exception.
A part from this, if you want your thread to return multiple values at different point of time then just pass multiple std::promise objects in thread and fetch multiple return values from thier associated multiple std::future objects.
Comentarios