Associative container in c++
- prashant raj
- Apr 4, 2021
- 4 min read
Updated: May 2, 2021
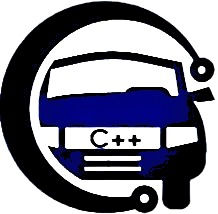
Associative containers implement sorted data structures that can be quickly searched.
Set:
Collection of unique keys, sorted by keys.
Sets are a type of associative containers in which each element has to be unique, because the value of the element identifies it. The value of the element cannot be modified once it is added to the set, though it is possible to remove and add the modified value of that element.
// Example program
#include<iostream>
#include<set>
using namespace std;
int main()
{
set<int> s1;
s1.insert(10);
s1.insert(50);
s1.insert(20);
s1.insert(40);
s1.insert(10);
s1.insert(30);
for(auto itr = s1.begin(); itr!= s1.end();itr++)
{
cout<<*itr<<endl;
}
return 0;
}
Output:
10
20
30
40
50
In above example we have added the value in unsorted order and value 10 added two times but in result we observed output came in sorted order and all the value are unique.
Member function in set
Insert():
pair<iterator,bool> insert (const value_type& val); pair<iterator,bool> insert (value_type&& val); with hint (2) iterator insert (const_iterator position, const value_type& val); iterator insert (const_iterator position, value_type&& val); range (3) template <class InputIterator> void insert (InputIterator first, InputIterator last); initializer list (4) void insert (initializer_list<value_type> il);
// Example program
#include<iostream>
#include<set>
using namespace std;
int main()
{
set<int> myset;
set<int>::iterator itr;
pair<set<int>::iterator,bool> ret;
for (int i=1; i<=5; ++i)
{
myset.insert(i*10);
}
ret = myset.insert(20); //value 20 was already in set so 20 is not added.
if (ret.second==false)
{
cout<<"Value 20 is already in set"<<endl;
itr=ret.first;
}
for(auto itr1 = itr; itr1!=myset.end();itr1++)
{
cout<<*itr1<<endl;
}
myset.insert (itr,25);
myset.insert (itr,20); // 20 already in set, not inserted
int myints[]= {5,10,15,20}; // 20 already in set, not inserted
myset.insert (myints,myints+3);
cout<<"DIsplay the main set"<<endl;
for(itr = myset.begin();itr!= myset.end();itr++)
{
cout<<*itr<<endl;
}
}
Find():
iterator find (const key_type& k);
const_iterator find (const key_type& k) const;
Get iterator to element
Searches the container for an element with a key equivalent to k and returns an iterator to it if found, otherwise it returns an iterator to set::end.
// Example program
#include<iostream>
#include<set>
using namespace std;
int main()
{
set<int> myset;
//set<int>::iterator itr;
for(int i=1 ; i<=5 ;i++)
{
myset.insert(10*i);
}
auto itr = myset.find(20);
if(itr != myset.end())
{
cout<<"Value is avilable in set"<<endl;
}
else
cout<<"Value is avilable in set"<<endl;
}
erase():
void erase (iterator position);
size_type erase (const value_type& val);
void erase (iterator first, iterator last);
Erase elements
Removes from the set container either a single element or a range of elements ([first,last)).
// Example program
#include<iostream>
#include<set>
using namespace std;
int main()
{
set<int> myset;
//set<int>::iterator itr;
for(int i=1; i<=10; i++)
{
myset.insert(10*i);
}
for(auto itr = myset.begin();itr!=myset.end();itr++)
{
cout<<*itr;
}
cout<<endl;
//find the value 50 and if avilable then remove from the set.
myset.erase(20);
auto itr = myset.find(50);
if(itr!=myset.end())
{
cout<<"Value find in set"<<endl;
//myset.erase(itr);
}
myset.erase(itr,myset.end());
for(auto itr = myset.begin();itr!=myset.end();itr++)
{
cout<<*itr;
}
cout<<endl;
}
Difference between push() and emplace():
push takes an existing element, and appends a copy of it to the container. Simple, straightforward. push always takes exactly one argument, the element to copy to the container.
emplace creates another instance of the class in the container, that's already appended to the container. The arguments to emplace are forwarded as arguments to the container's class's constructor. Emplace can have either one argument, more than one argument, or no argument at all, if the class has a default constructor.
Map in C++:
Maps are associative containers that store elements in a mapped fashion. Each element has a key value and a mapped value. No two mapped values can have same key values.
In a map, the key values are generally used to sort and uniquely identify the elements, while the mapped values store the content associated to this key. The types of key and mapped value may differ, and are grouped together in member type value_type, which is a pair type combining both:
typedef pair<const Key, T> value_type;
*Maps are typically implemented as binary search trees
Member function in Map:
Insert():
single element (1)
pair<iterator,bool> insert (const value_type& val);
with hint (2)
iterator insert (iterator position, const value_type& val);
range (3)
template <class InputIterator>
void insert (InputIterator first, InputIterator last);
#include<iostream>
#include<map>
using namespace std;
int main()
{
map <char,int> mymap;
mymap.insert(pair<char,int>('a',20));
mymap.insert(pair<char,int>('b',45));
map<char, int>::iterator itr;
for(itr = mymap.begin(); itr!= mymap.end(); itr++)
{
cout<<itr->first << " "<< itr->second <<endl;
}
pair<std::map<char,int>::iterator,bool> ret;
ret = mymap.insert ( std::pair<char,int>('b',500) );
if (ret.second==false) {
std::cout << "element 'b' already existed";
std::cout << " with a value of " << ret.first->second << '\n';
}
itr = mymap.begin();
mymap.insert(itr,pair<char,int>('c',35));
mymap.insert(itr,pair<char,int>('d',45));
for(itr = mymap.begin(); itr!= mymap.end(); itr++)
{
cout<<itr->first << " "<< itr->second <<endl;
}
cout<<"In another map" <<endl;
map<char,int> anothermap;
anothermap.insert(mymap.begin(),mymap.find('c'));
for(itr = anothermap.begin(); itr!= anothermap.end(); itr++)
{
cout<<itr->first << " "<< itr->second <<endl;
}
return 0;
}
output:
a 20
b 45
element 'b' already existed with a value of 45
a 20
b 45
c 35
d 45
In another map
a 20
b 45
Find():
iterator find (const key_type& k);
const_iterator find (const key_type& k) const;
Get iterator to element
Searches the container for an element with a key equivalent to k and returns an iterator to it if found, otherwise it returns an iterator to map::end.
#include<iostream>
#include<map>
using namespace std;
int main()
{
map<char,int> mymap;
mymap.insert(pair<char,int>('a',25));
mymap.insert(pair<char,int>('b',35));
mymap.insert(pair<char,int>('c',15));
mymap.insert(pair<char,int>('d',10));
map<char,int>::iterator itr;
itr = mymap.find('c');
if(itr != mymap.end())
{
mymap.erase(itr);
}
for(itr = mymap.begin(); itr!= mymap.end();itr++)
{
cout<<itr->first<<" "<<itr->second<<endl;
}
}
Output:
a 25
b 35
d 10
erase()
iterator erase (const_iterator position);
size_type erase (const key_type& k);
iterator erase (const_iterator first, const_iterator last);
Erase elements
Removes from the map container either a single element or a range of elements ([first,last)).
#include<iostream>
#include<map>
using namespace std;
int main()
{
map <char,int> mymap;
mymap.insert(pair<char,int>('a',25));
mymap.insert(pair<char,int>('k',25));
mymap.insert(pair<char,int>('p',25));
mymap.insert(pair<char,int>('l',25));
mymap.insert(pair<char,int>('b',35));
mymap.insert(pair<char,int>('c',40));
mymap.insert(pair<char,int>('d',30));
mymap.insert(pair<char,int>('e',30));
mymap.insert(pair<char,int>('f',30));
mymap.insert(pair<char,int>('g',30))
mymap.erase('c');
map<char,int>::iterator itr;
itr = mymap.find('b');
mymap.erase(itr);
itr = mymap.find('g');
mymap.erase(itr,mymap.end());
for(itr = mymap.begin();itr!= mymap.end();itr++)
{
cout<<itr->first<<" "<<itr->second<<endl;
}
}
Comments